This patches implements the auto keyword from the N3007 standard
specification.
This allows deducing the type of the variable like in C++:
```
auto nb = 1;
auto chr = 'A';
auto str = "String";
```
The list of statements which allows the usage of auto:
* Basic variables declarations (int, float, double, char, char*...)
* Macros declaring a variable with the auto type
The list of statements which will not work with the auto keyword:
* auto arrays
* sizeof(), alignas()
* auto parameters, auto return type
* auto as a struct/typedef member
* uninitialized auto variables
* auto in an union
* auto as a enum type specifier
* auto casts
* auto in an compound literals
Differential Revision: https://reviews.llvm.org/D133289
This fixes many unit tests when trying to enable IncrementalExtensions
by default for testing purposes.
Differential Revision: https://reviews.llvm.org/D158415
The bounds of a c++ array is a _constant-expression_. And in C++ it is
also a constant expression.
But we also support VLAs, ie arrays with non-constant bounds.
We need to take care to handle the case of a consteval function (which
are specified to be only immediately called in non-constant contexts)
that appear in arrays bounds.
This introduces `Sema::isAlwayConstantEvaluatedContext`, and a flag in
ExpressionEvaluationContextRecord, such that immediate functions in
array bounds are always immediately invoked.
Sema had both `isConstantEvaluatedContext` and
`isConstantEvaluated`, so I took the opportunity to cleanup that.
The change in `TimeProfilerTest.cpp` is an unfortunate manifestation of
the problem that #66203 seeks to address.
Fixes#65520
This new method repeatedly calls Lex() until end of file is reached
and optionally fills a std::vector of Tokens. Use it in Clang's unit
tests to avoid quite some code duplication.
Differential Revision: https://reviews.llvm.org/D158413
with an explicit parameter.
We tried to read a pointer to a non-existent `This` APValue when
constant-evaluating an explicit object lambda call operator (the `this`
pointer is never set in explicit object member functions)
Fixes#68070
This reverts commit a1e81d2ead.
Revert "Fix test hip-offload-compress-zlib.hip"
This reverts commit ba01ce6066.
Revert due to sanity fail at
https://lab.llvm.org/buildbot/#/builders/5/builds/37188https://lab.llvm.org/buildbot/#/builders/238/builds/5955
/b/sanitizer-aarch64-linux-bootstrap-ubsan/build/llvm-project/clang/lib/Driver/OffloadBundler.cpp:1012:25: runtime error: load of misaligned address 0xaaaae2d90e7c for type 'const uint64_t' (aka 'const unsigned long'), which requires 8 byte alignment
0xaaaae2d90e7c: note: pointer points here
bc 00 00 00 94 dc 29 9a 89 fb ca 2b 78 9c 8b 8f 77 f6 71 f4 73 8f f7 77 73 f3 f1 77 74 89 77 0a
^
#0 0xaaaaba125f70 in clang::CompressedOffloadBundle::decompress(llvm::MemoryBuffer const&, bool) /b/sanitizer-aarch64-linux-bootstrap-ubsan/build/llvm-project/clang/lib/Driver/OffloadBundler.cpp:1012:25
#1 0xaaaaba126150 in clang::OffloadBundler::ListBundleIDsInFile(llvm::StringRef, clang::OffloadBundlerConfig const&) /b/sanitizer-aarch64-linux-bootstrap-ubsan/build/llvm-project/clang/lib/Driver/OffloadBundler.cpp:1089:7
Will reland after fixing it.
Commit 9370271ec5 made HashBuilder an
alias for HashBuilderImpl:
template <class HasherT, support::endianness Endianness>
using HashBuilder = HashBuilderImpl<HasherT, Endianness>;
This patch renames HashBuilderImpl to HashBuilder while removing the
alias above.
The 'counted_by' attribute is used on flexible array members. The
argument for the attribute is the name of the field member in the same
structure holding the count of elements in the flexible array. This
information can be used to improve the results of the array bound sanitizer
and the '__builtin_dynamic_object_size' builtin.
This example specifies the that the flexible array member 'array' has the
number of elements allocated for it in 'count':
struct bar;
struct foo {
size_t count;
/* ... */
struct bar *array[] __attribute__((counted_by(count)));
};
This establishes a relationship between 'array' and 'count', specifically
that 'p->array' must have *at least* 'p->count' number of elements available.
It's the user's responsibility to ensure that this relationship is maintained
through changes to the structure.
In the following, the allocated array erroneously has fewer elements than
what's specified by 'p->count'. This would result in an out-of-bounds access not
not being detected:
struct foo *p;
void foo_alloc(size_t count) {
p = malloc(MAX(sizeof(struct foo),
offsetof(struct foo, array[0]) + count *
sizeof(struct bar *)));
p->count = count + 42;
}
The next example updates 'p->count', breaking the relationship requirement that
'p->array' must have at least 'p->count' number of elements available:
struct foo *p;
void foo_alloc(size_t count) {
p = malloc(MAX(sizeof(struct foo),
offsetof(struct foo, array[0]) + count *
sizeof(struct bar *)));
p->count = count + 42;
}
void use_foo(int index) {
p->count += 42;
p->array[index] = 0; /* The sanitizer cannot properly check this access */
}
Reviewed By: nickdesaulniers, aaron.ballman
Differential Revision: https://reviews.llvm.org/D148381
Enables or disables verification of the generated LLVM IR.
Users can pass this to turn on extra verification to catch certain types of
compiler bugs at the cost of extra compile time.
All of the _Builtin_stdarg and _Builtin_stddef submodules need to be
allowed from [no_undeclared_includes] modules. Split the builtin headers
tests out from the compiler_builtins test so that the testing modules
can be modified without affecting the other many tests that use
Inputs/System/usr/include.
Since 78d649a417 the recommended way to
pass an executor is to use the _TEST_PARAMS variable, which means we now
pass more complicated value (including ones that may contain multiple
`=`) as part of this variable. However, the `REGEX REPLACE` being used
has greedy matches so everything up to the last = becomes part of the
variable name which results in invalid syntax in the generated lit
config file.
This was noticed due to builder failures for those using the
CrossWinToARMLinux.cmake cache file.
---------
Co-authored-by: Vladimir Vereschaka <vvereschaka@accesssoftek.com>
Summary:
Weak symbols are supposed to have the semantics that they can be
overriden by a strong (i.e. global) definition. This wasn't being
respected by the LTO pass because we simply used the first definition
that was available. This patch fixes that logic by doing a first pass
over the symbols to check for strong resolutions that could override a
weak one.
A lot of fake linker logic is ending up in the linker wrapper. If there
were an option to handle this in `lld` it would be a lot cleaner, but
unfortunately supporting NVPTX is a big restriction as their binaries
require the `nvlink` tool.
If there are two guides, one of them generated from a non-templated
constructor
and the other from a templated constructor, then the standard gives
priority to
the first. Clang detected ambiguity before, now the correct guide is
chosen.
The correct behavior is described in this paper:
http://wg21.link/P0620R0
Example for the bug: http://godbolt.org/z/ee3e9qG78
As an unrelated minor change, fix the issue
https://github.com/llvm/llvm-project/issues/64020,
which could've led to incorrect behavior if further development inserted
code after a call to
`isAddressSpaceSubsetOf()`, which specified the two parameters in the
wrong order.
---------
Co-authored-by: hobois <horvath.botond.istvan@gmial.com>
This test fails as .eh_frame handling is not yet implemented for RISC-V
in JITLink. #66067 is proposed to address this.
Skip the test until the issue is resolved. It seems that D159167 enabled
this test for more than just ppc64. As the test always failed, it just
wasn't run until now, I think skipping is the correct interim approach
(as is already done for Arm, Darwin, and others).
Add option -f[no-]offload-compress to clang to enable/disable
compression of device binary for HIP. By default it is disabled.
Add option -compress to clang-offload-bundler to enable compression of
offload bundle. By default it is disabled.
When enabled, zstd or zlib is used for compression when available.
When disabled, it is NFC compared to previous behavior. The same offload
bundle format is used as before.
Clang-offload-bundler automatically detects whether the input file to be
unbundled is compressed and the compression method and decompress if
necessary.
I've eliminated the `logText("Block converged")` call entirely because
a) These logs are associated with an individual `CFGElement`, while
convergence
should be associated with a block, and
b) The log message was being associated with the wrong block:
`recordState()`
dumps all of the pending log messages, but `blockConverged()` is called
after
the last `recordState()` call for a given block, so that the "Block
converged" log message was being associated with the first element of
the
_next_ block to be processed.
Example:
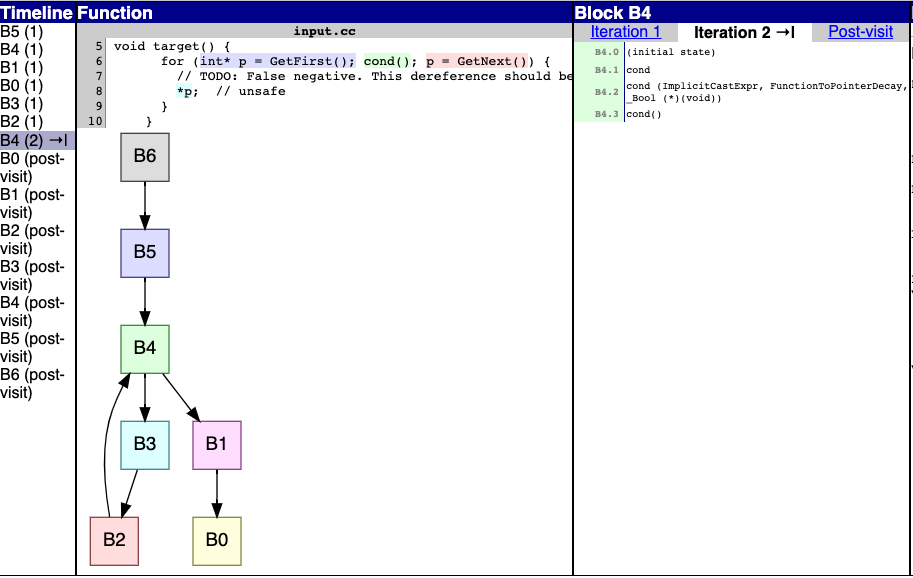
D63960 performs a tree transformation on AST to prevent evaluating
constant expressions eagerly by removing recorded immediate consteval
invocations from subexpressions. (See
https://reviews.llvm.org/D63960#inline-600736 for its motivation.)
However, the UDL node has been replaced with a CallExpr in the default
TreeTransform since ca844ab0 (inadvertently?). This confuses clangd as
it relies on the exact AST node type to decide whether or not to present
inlay hints for an expression.
With regard to the fix, I think it's enough to return the UDL expression
as-is during the transformation: We've bound it to temporary in its
construction, and there's no ConstantExpr to visit under a UDL.
Fixes https://github.com/llvm/llvm-project/issues/63898.
Reland 6a621ed8e4 which failed on Windows but not macOS.
The failures were caused by moving the annotation of the children from the
top to the bottom of TokenAnnotator::annotate(), resulting in invalid lines
including incomplete ones being skipped.
This patch fixes the display of characters appearing in LHS or RHS of == expression in notes to static assertion failure.
This applies C-style escape if the printed character is a special character. This also adds a numerical value displayed next to the character representation.
This also tries to print multi-byte characters if the user-provided expression is multi-byte char type.
Reviewed By: cor3ntin
Differential Revision: https://reviews.llvm.org/D155610
Instantiating a lambda at a scope different from where it is defined
will paralyze clang if the trailing require clause refers to local
variables. This patch fixes this by re-adding the local variables to
`LocalInstantiationScope`.
Fixes#64462
After annotating constructors/destructors as FunctionDeclarationName in
commit 0863051208, we have seen several issues because ctors/dtors had
been treated differently than functions in aligning, wrapping, and
indenting.
This patch annotates ctors/dtors as CtorDtorDeclName instead and would
effectively revert commit 0468fa07f8, which is obsolete now.
Fixed#67903.
Fixed#67907.
```
@interface A : NSObject
@property (nonnull, nonatomic, strong) NSString *name;
+ (nullable instancetype)shared;
@end
@[[A shared].name];
```
Consider the code above, the nullability of the name property should
depend on the result of the shared method. A warning is expected because
of adding a nullable object to array.
ObjCMessageExpr gets the actual type through
Sema::getMessageSendResultType, instead of using the return type of
MethodDecl directly. The final type is generated by considering the
nullability of receiver and MethodDecl together.
Thus, the RetType in NullabilityChecker should all be replaced with
M.getOriginExpr()->getType().
Co-authored-by: tripleCC <triplecc@gmail.com>
This way, it the rtti_proxies can be candidates for being replaced
altogether with GOTPCREL relocations because they are discardable.
Functionally, this shouldn't change the final ELF linkage of the
proxies.
…on and initialization.
The patch implements FEAT_LRCPC3 support (Load-Acquire RCpc instructions
version 3) in Function Multi Versioning. To maintain compatibility while
features list grows extension bit FEAT_EXT and initialization bit
FEAT_INIT are reserved.
This patch removes the `SourceManager` APIs that create `FileID` from a
`const FileEntry *` in favor of APIs that take `FileEntryRef`. This also
removes a misleading documentation that claims `nullptr` file entry
represents stdin. I don't think that's right, since we just try to
dereference that pointer anyways.
Make top level modules for all the C standard library headers.
The `__stddef` implementation headers need header guards now that they're all modular. stdarg.h and stddef.h will be textual headers in the builtin modules, and so need to be repeatedly included in both the system and builtin module case. Define their header guards for consistency, but ignore them when building with modules.
`__stddef_null.h` needs to ignore its header guard when modules aren't being used to fulfill its redefinition obligation.
`__stddef_nullptr_t.h` needs to add a guard for C23 so that `_Builtin_stddef` can compile in C17 and earlier modes. `_Builtin_stddef.nullptr_t` can't require C23 because it also needs to be usable from C++.
Reviewed By: Bigcheese
Differential Revision: https://reviews.llvm.org/D159064